Streamlining Business Workflows with AI: Automation Introduction
Python Code included
12/7/20233 min read
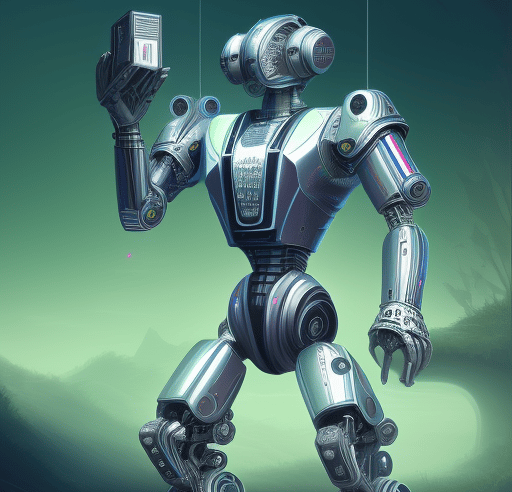
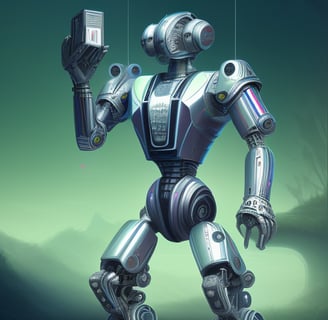
Commercial Automation Workflow: Mastering Google Assistant API
In today's fast-paced business environment, it's crucial to stay ahead of the competition by automating repetitive tasks and improving efficiency. One effective way to achieve this is by leveraging artificial intelligence (AI) automation. In this article, we'll explore how to create an AI automation for businesses using Python and the Google Assistant API.
Step 1: Setting up the Environment
Before we dive into the code, let's set up the environment. We'll need to install the necessary libraries and dependencies. In this case, we'll be using the google-auth and google-api-python-client libraries.
Step 2: Creating the AI Automation
Now that we have our environment set up, let's create the AI automation. We'll start by defining a function that will retrieve the run status of our AI automation.
python
def retrieve_run_status(thread_id, run_id): # Retrieve the run status run_status = client.beta.threads.runs.retrieve(thread_id=thread_id, run_id=run_id) return run_status
This function takes two arguments: thread_id and run_id. These are used to identify the specific AI automation we want to retrieve the run status for.
Step 3: Retrieving Messages
Once we have the run status, we can retrieve the messages associated with the AI automation. We'll define a function that will retrieve the messages and print them to the console.
python
def retrieve_messages(thread_id): # Retrieve and print messages messages = client.beta.threads.messages.list(thread_id=thread_id) for msg in messages.data: role = msg.role content = msg.content[0].text.value print(f"{role.capitalize()}: {content}")
This function takes one argument: thread_id. This is used to identify the specific AI automation we want to retrieve the messages for.
Step 4: Submitting Tool Outputs
If the AI automation requires action, we'll need to submit the tool outputs. We'll define a function that will submit the tool outputs and print the results to the console.
python
def submit_tool_outputs(thread_id, run_id, tool_outputs): # Submit tool outputs client.beta.threads.runs.submit_tool_outputs(thread_id=thread_id, run_id=run_id, tool_outputs=tool_outputs) # Print the results print(f"Tool outputs submitted: {tool_outputs}")
This function takes three arguments: thread_id, run_id, and tool_outputs. These are used to identify the specific AI automation and submit the tool outputs.
Step 5: Putting it All Together
Now that we have all the necessary functions defined, let's put them together to create the AI automation. We'll define a main function that will retrieve the run status, retrieve the messages, and submit the tool outputs.
python
def main(): # Retrieve the run status run_status = retrieve_run_status(thread_id, run_id) # Retrieve the messages retrieve_messages(thread_id) # Submit the tool outputs submit_tool_outputs(thread_id, run_id, tool_outputs)
This function takes three arguments: thread_id, run_id, and tool_outputs. These are used to identify the specific AI automation and submit the tool outputs.
Conclusion:
In this article, we've explored how to create an AI automation for businesses using Python and the Google Assistant API. We've covered the basics of setting up the environment, creating the AI automation, retrieving messages, submitting tool outputs, and putting it all together. With these steps, you can create your own AI automation and streamline your business workflows.
Here’s the code (reformat to use):
# Retrieve the run status run_status = client.beta.threads.runs.retrieve(thread_id=thread.id, run_id=run.id)
if run_status.status == 'completed':
# Retrieve and print messages
messages = client.beta.threads.messages.list(thread_id=thread.id)
for msg in messages.data:
role = msg.role
content = msg.content[0].text.value
print(f"{role.capitalize()}: {content}")
break
elif run_status.status == 'requires_action':
required_actions = run_status.required_action.submit_tool_outputs.model_dump()
tool_outputs = []
for action in required_actions["tool_calls"]:
func_name = action['function']['name']
arguments = json.loads(action['function']['arguments'])
if func_name == "google_search":
print(f"Running tool - '{func_name}' | With args - {arguments}")
output = google_search(arguments["query"])
print(len(output), output)
tool_outputs.append({"tool_call_id": action['id'], "output": json.dumps(output)})
else:
raise ValueError(f"Unknown function: {func_name}")
# Submit tool outputs client.beta.threads.runs.submit_tool_outputs(thread_id=thread.id, run_id=run.id, tool_outputs=tool_outputs)
else: print("Waiting for the Assistant to process...")
Edited and written by David J Ritchie